Noise
- maiguzman28
- Jul 14, 2020
- 4 min read
Updated: Jul 16, 2020
Well, it happened... there is no way around it, so I had to learn at least the basics of noise. In the fun coding session, that was not fun at all to me, I did not understand anything about this topic. So I decided to go back to the tutorials of Daniel S. to see if maybe that way I can understand it. Wish me luck...
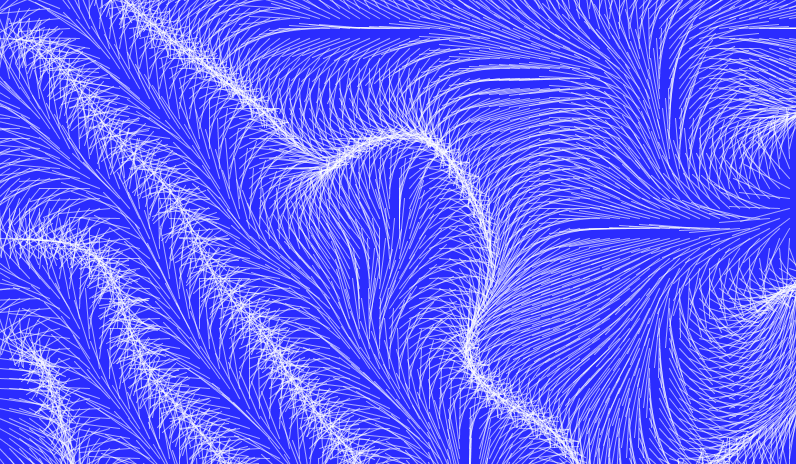
Noise allow us to create a smooth randomness, much more organic. In theory, I like this a lot, because it will give me the possibility to design more smoothly.
This is something very important about noise: for random it is very clear what the arguments to that functions are: minum and maximum. The noise function only give us a value between 0 and 1!!!
Processing has a built-in implementation of the Perlin noise algorithm with the function noise( ). The noise( ) function takes one, two, or three arguments (referring to the “ space ” in which noise is computed: one, two, or three dimensions).
One-dimensional Perlin noise produces as a linear sequence of values over time. For example: 0.364, 0.363, 0.363, 0.364, 0.365
In order to get these numbers out of Processing, we have to do two things:
(1) call the function noise( ), and
(2) pass in as an argument the current “ time. ” We would typically start at time t 0 and therefore call the function like so: “ noise(t); ”
The noise value is scaled by multiplying by the width of the window. If the width is 200, and the range of noise( ) is between 0.0 and 1.0, the range of noise( ) multiplied by the width is 0.0 to 200.0. This is illustrated by the table below
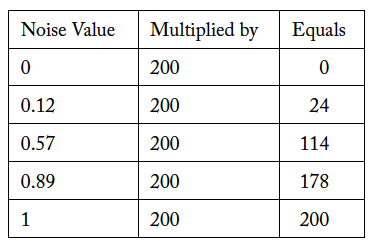
Aaaaah fiiiinally some light... To know that there was a small chapter in the book about noise would have been very helpful. It is the first time I feel like I started to understand! Yeay!
Exercise 13-3: Complete the following code which uses Perlin noise to set the location of
a circle. Run the code. Does the circle appear to be moving “ naturally ” ?
Here is the code:
float xtime = 0.0;
float ytime = 100.0;
float increment = 0.01;
void setup() {
size(200, 200);
smooth();
}
void draw() {
background(0);
float x = noise(xtime) * width;
float y = noise(ytime) * height;
xtime += increment;
ytime += increment;
// Draw the ellipse with size determined by Perlin noise
noStroke();
fill(200);
ellipse(x, y, 20, 20);
}
This I finally understood!!!
So I'm going to start doing the script excercises, wish me luck!
The first one was not that complicated, once you understand what you are doing:
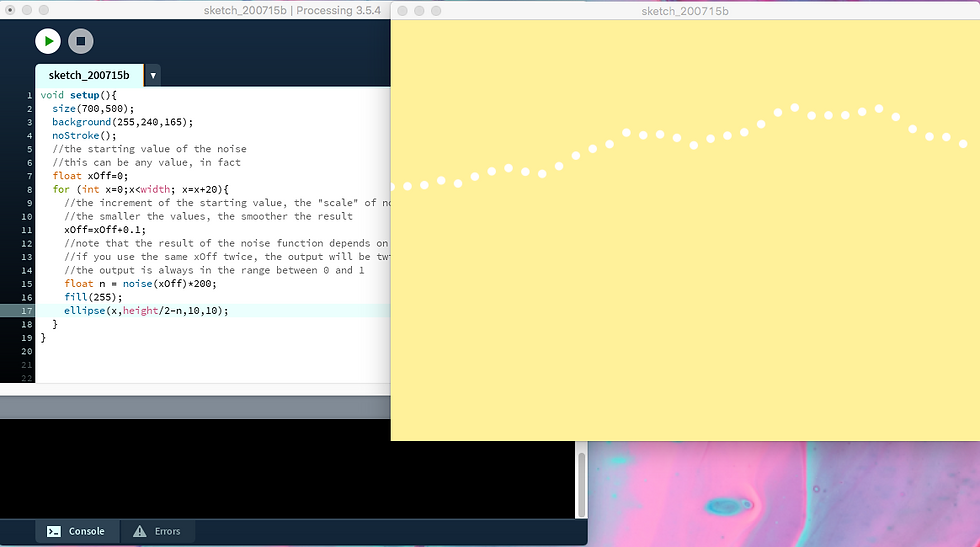
Playing a little bit with the increment value made this:
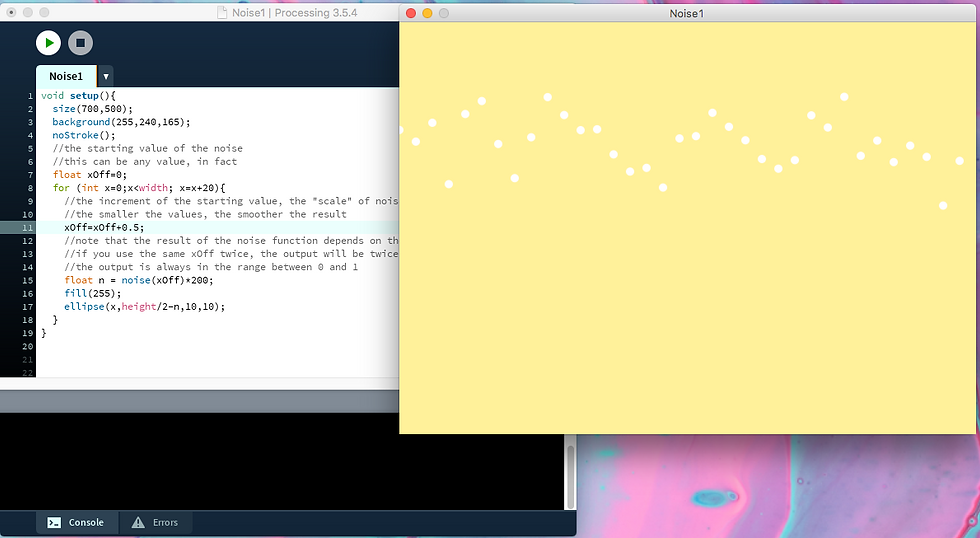
This looks more random. You can still see an intention behind the positions, but it is not that clear as when the value is lower. Oh, ok.
If I do this with my corona instead of the ellipses it looks like this:

Kinda disgusting... If I decrease the value from xOff+0.5 to xOff+0.05; it looks like this:
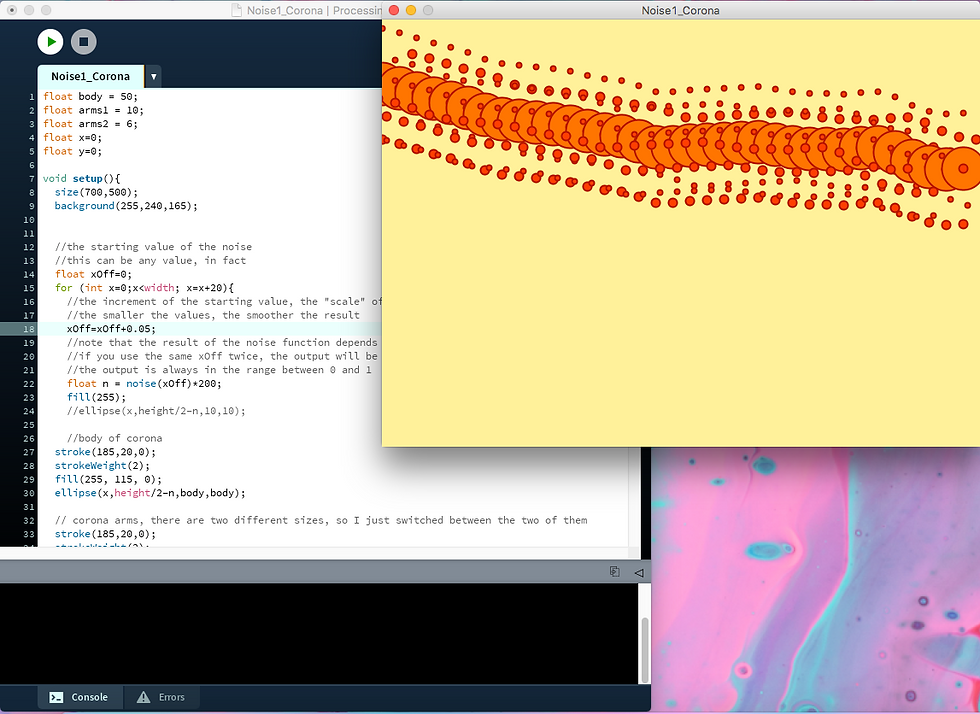
float body = 50;
float arms1 = 10;
float arms2 = 6;
float x=0;
float y=0;
void setup(){
size(700,500);
background(255,240,165);
//the starting value of the noise
//this can be any value, in fact
float xOff=0;
for (int x=0;x<width; x=x+20){
//the increment of the starting value, the "scale" of noise
//the smaller the values, the smoother the result
xOff=xOff+0.05;
//note that the result of the noise function depends on the input value xOff
//if you use the same xOff twice, the output will be twice the same
//the output is always in the range between 0 and 1
float n = noise(xOff)*200;
fill(255);
//ellipse(x,height/2-n,10,10);
//body of corona
stroke(185,20,0);
strokeWeight(2);
fill(255, 115, 0);
ellipse(x,height/2-n,body,body);
// corona arms, there are two different sizes, so I just switched between the two of them
stroke(185,20,0);
strokeWeight(2);
fill(255,60,0);
ellipse(x,height/2-n,arms1,arms1);
ellipse(x-25,height/2-n-15,arms2,arms2);
ellipse(x-35,height/2-n+27,arms1,arms1);
ellipse(x+30,height/2-n+15,arms2,arms2);
ellipse(x+35,height/2-n-35,arms1,arms1);
ellipse(x+45,height/2-n+45,arms2,arms2);
ellipse(x-65,height/2-n,arms1,arms1);
ellipse(x-35,height/2-n+55,arms2,arms2);
ellipse(x,height/2-n+65,arms1,arms1);
ellipse(x,height/2-n-65,arms2,arms2);
ellipse(x+65,height/2-n,arms1,arms1);
ellipse(x-45,height/2-n-45,arms2,arms2);
}
}
Till now, it was one dimensional noise. If we think twodimensional, we can create kind of like clouds. I had fun with the code and started to try some things:
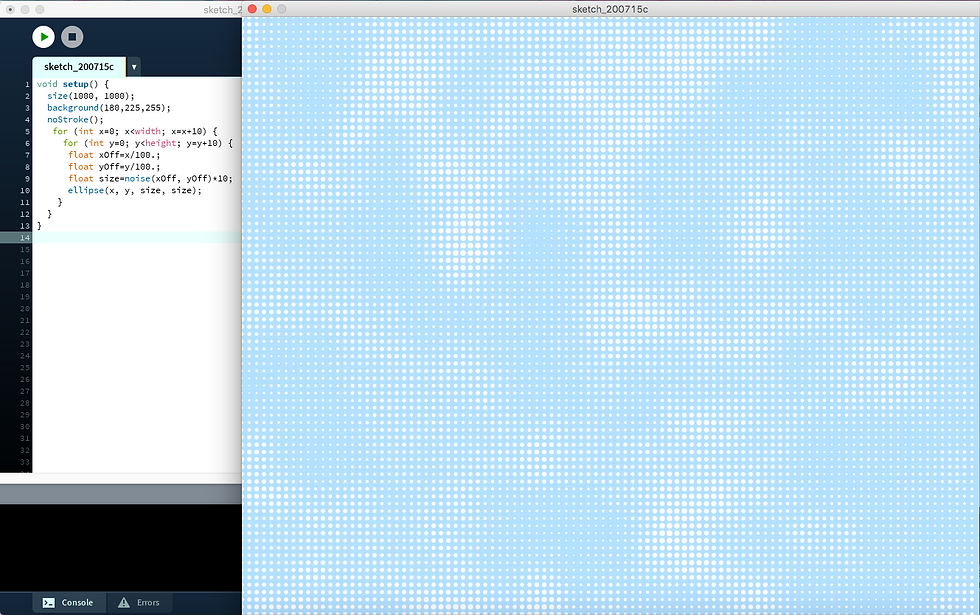
If I increment the value to *30 instead of *10 this happens:
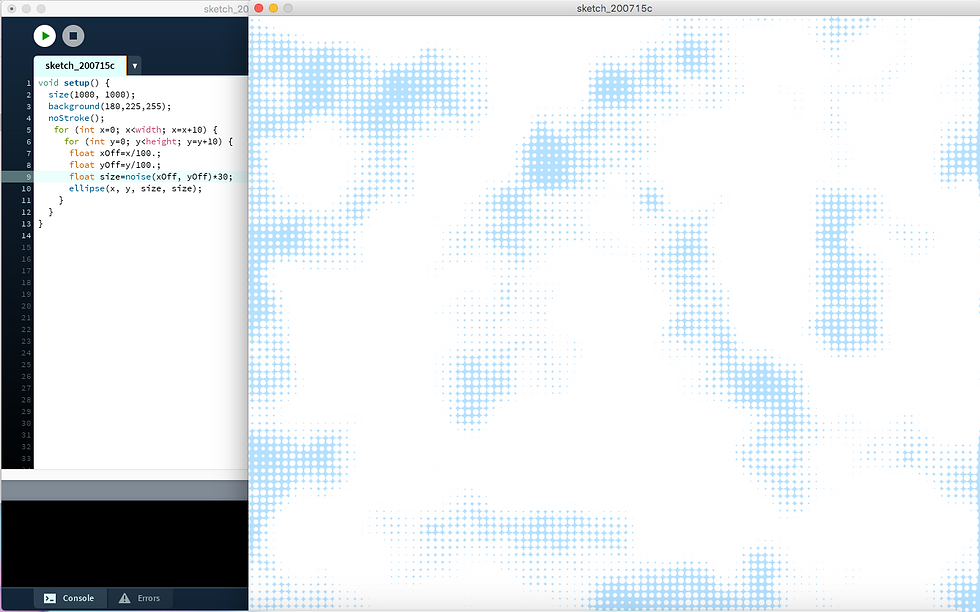
I then made outlines and multuply it by *100

It looks like a weird texture. Then I multiply it by *50 and also changed the float xOff and yOff dividing it by 10 and not 100, like the las example:
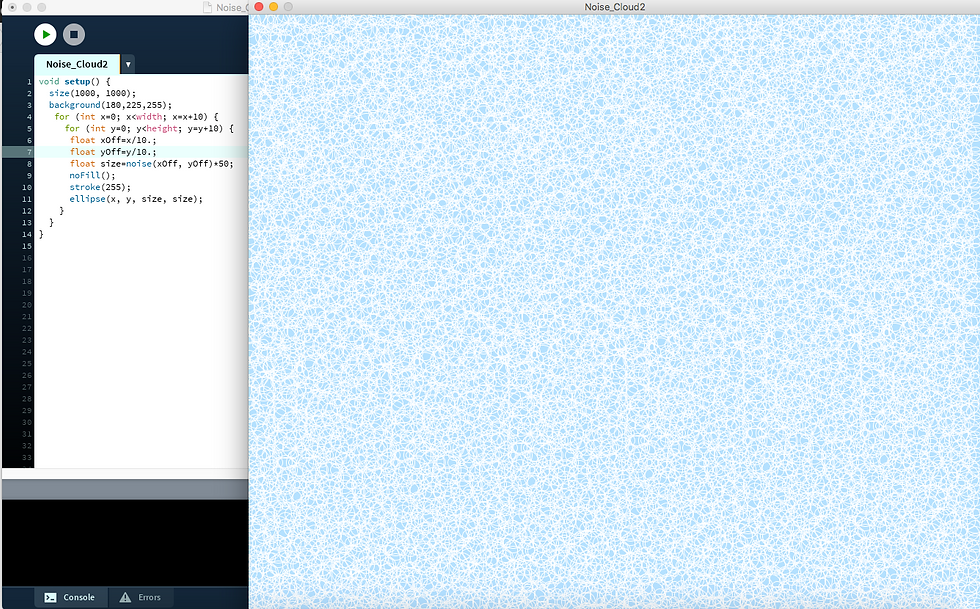
Finally I multiplied the value *10 instead of *50, to give everything a little room. I did that with outlines, this looks like bubbles and I did that with ellipses:
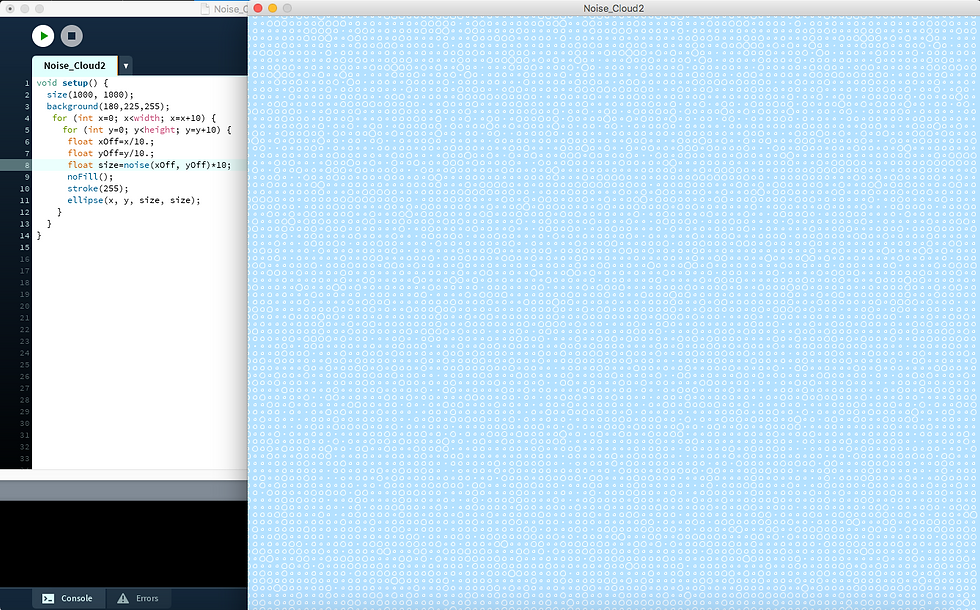

Now I tried this with my Corona:
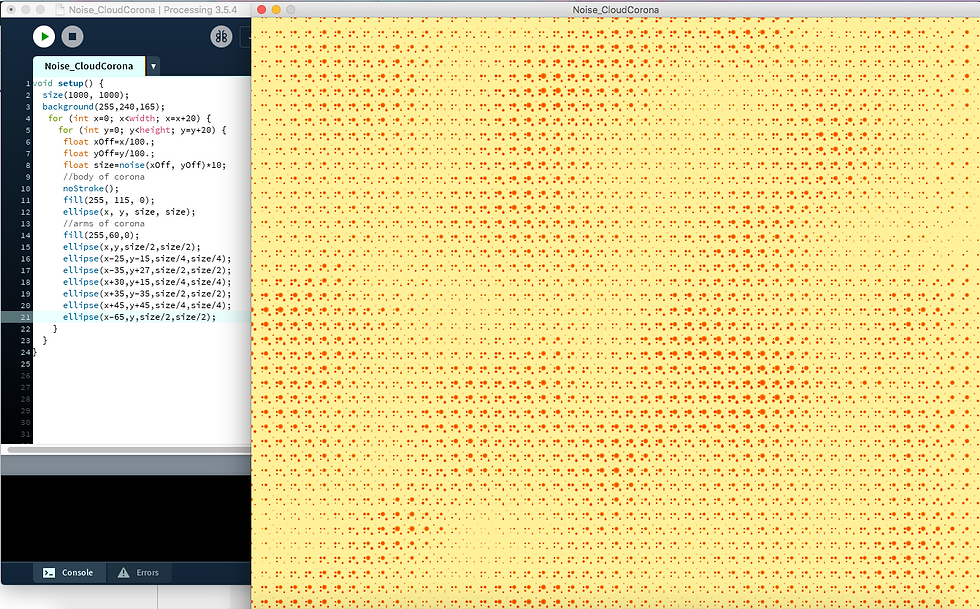
It needs a little bit of tuning. I founded an easier way to use this on the script. It is the modularized version of it, and I did a drawCorona function. This made it easier, since I need the noiseFactor to every element of my corona, body and arms:

This is the code:
void setup() {
size(1000, 1000);
background(255,240,165);
for (int x=0; x<width; x=x+10) {
for (int y=0; y<height; y=y+10) {
float noiseFactor=noise(x/100., y/100.)*10;
drawCorona(x,y,noiseFactor);
}
}
}
void drawCorona(float x, float y, float noiseFactor){
//body of corona
noStroke();
fill(255, 115, 0);
ellipse(x, y, noiseFactor, noiseFactor);
//arms of corona
fill(255,60,0);
ellipse(x,y,noiseFactor/2,noiseFactor/2);
ellipse(x-25,y-15,noiseFactor/4,noiseFactor/4);
ellipse(x-35,y+27,noiseFactor/2,noiseFactor/2);
ellipse(x+30,y+15,noiseFactor/4,noiseFactor/4);
ellipse(x+35,y-35,noiseFactor/2,noiseFactor/2);
ellipse(x+45,y+45,noiseFactor/4,noiseFactor/4);
ellipse(x-65,y,noiseFactor/2,noiseFactor/2);
}
And the input flow never stops: Now we have to learn and work with rotating. I found this on the processing website and I think this is very important to understand:
pushMatrix() is a built-in function that saves the current position of the coordinate system. The translate(60, 80) moves the coordinate system 60 units right and 80 units down. The rect(20, 20, 40, 40) draws the rectangle at the same place it was originally. Remember, the things you draw don’t move—the grid moves instead. Finally, popMatrix() restores the coordinate system to the way it was before you did the translate.
I got the code from the script and tried it:
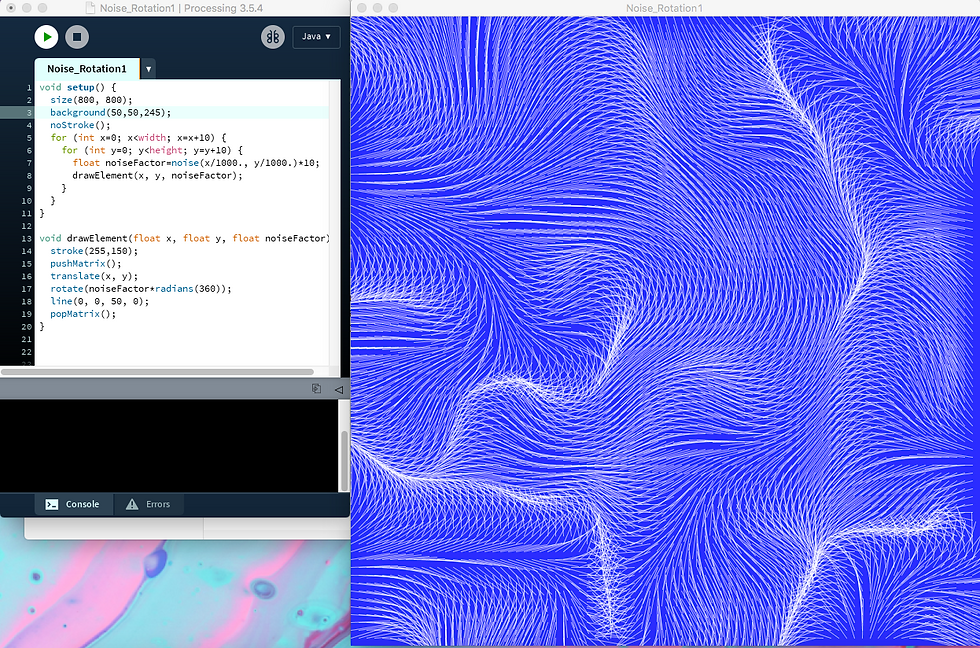
I increased the noise factor and multiplied it by *100 instead of *10 and this happened:
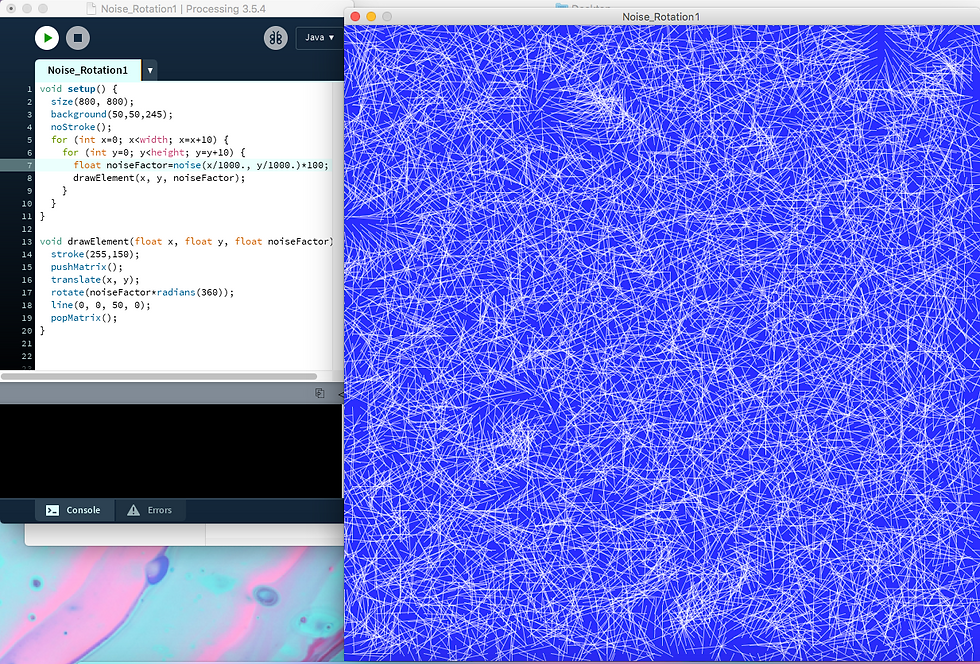
Comments