Trigonometry 2.0
- maiguzman28
- Jul 14, 2020
- 3 min read
Updated: Jul 16, 2020
Since I had so many problems with Trigonometry, I decided to do more excercises on that, because I don't really feel like I fully understand it. So I am starting with the basics again.

The first excercise was to draw a circle using Trigonometry:
The code was very simple and how it works is, that using cos and sin, we increment the angle on theta, this way the location is updated and looks like a circle being drawn. Playing with the number (+= 0.04) allows the circles to be closer or more separate to one another, this way it looks like a closed circle being drawn, or a lot of circles, like this (+= 3) :
If we increment not only the angle, but also the radius of the ellipse, this happens:
This looks a little like a candy.
So I went on in trying the excercises from the script again, and go through the code, in order to understand it completely:

This is the code:
void setup() {
size(400, 400);
background(255,240,245);
//this is the big circle, placed in the middle of the window
noStroke();
ellipse(200, 200, 40, 40);
for (int a=0; a<360; a=a+40) This allows you to play with the distance between the small circles and because of their distance, less or more circles appear {
//those are the small circles around the big circle
//to calculate their positions, I use "cos" and "sin"
int dx = (int)(cos(radians(a))*30); This (the *30 value) defines the distance from the small circles to the center, if the value is bigger, the distance to the center of the big circle is bigger
int dy = (int)(sin(radians(a))*30);
fill(255,185,195);
ellipse(200+dx, 200+dy, 9, 9);This is where the cos and sin value come in handy. The position is 200 and 200, which is the middle of the window, and then I added the cos and sin value making it 200+dx and 200+dy
}
}
The next one was to put the circles around the big circle and multiply them:
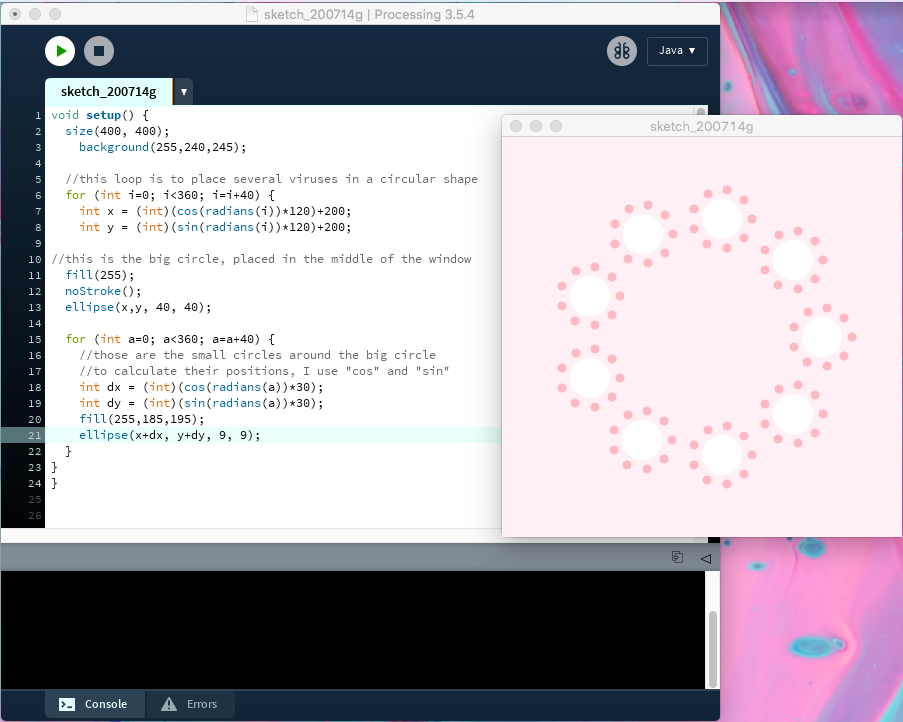
This is the code:
void setup() {
size(400, 400);
background(255,240,245);
//this loop is to place several viruses in a circular shape
for (int i=0; i<360; i=i+40) This value determines the distance between the groups of circles {
int x = (int)(cos(radians(i))*120)+200; The *120 determines the distance to the center and the +200 makes the middle of the window, the certer
int y = (int)(sin(radians(i))*120)+200;
//this is the big circle, placed in the middle of the window
fill(255);
noStroke();
ellipse(x,y, 40, 40);
for (int a=0; a<360; a=a+40) {
//those are the small circles around the big circle
//to calculate their positions, I use "cos" and "sin"
int dx = (int)(cos(radians(a))*30);
int dy = (int)(sin(radians(a))*30);
fill(255,185,195);
ellipse(x+dx, y+dy, 9, 9);This makes the position of the circles x, which is already being calculated on the top and adds the dx and dy
}
}
}
This is the next excercise of Trigonometry:
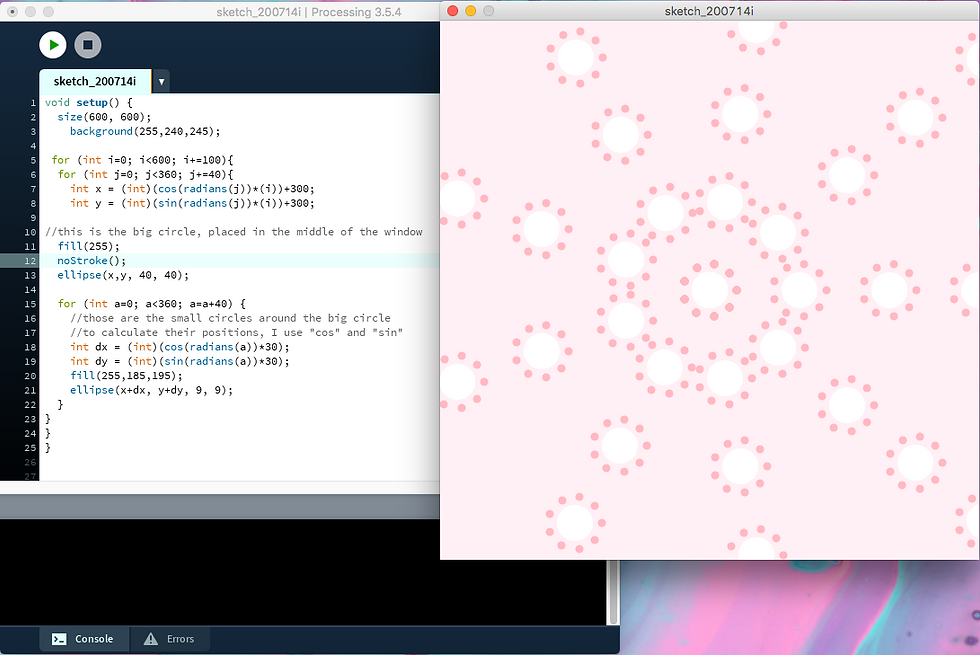
Here we have two important aspects: i and j.
void setup() {
size(600, 600);
background(255,240,245);
for (int i=0; i<600; i+=100)This is for the distances between the groups of circles{
for (int j=0; j<360; j+=40)This is for the distance between the groups of circles inside a circle. If we increment the value, the distance or arms of the circles are way less, if we increase the value, there are more arms{
int x = (int)(cos(radians(j))*(i))+300;
int y = (int)(sin(radians(j))*(i))+300;
//this is the big circle, placed in the middle of the window
fill(255);
noStroke();
ellipse(x,y, 40, 40);
for (int a=0; a<360; a=a+40) {
//those are the small circles around the big circle
//to calculate their positions, I use "cos" and "sin"
int dx = (int)(cos(radians(a))*30);
int dy = (int)(sin(radians(a))*30);
fill(255,185,195);
ellipse(x+dx, y+dy, 9, 9);
}
}
}
}
So this was the last of the trigonometry excercises I did again:
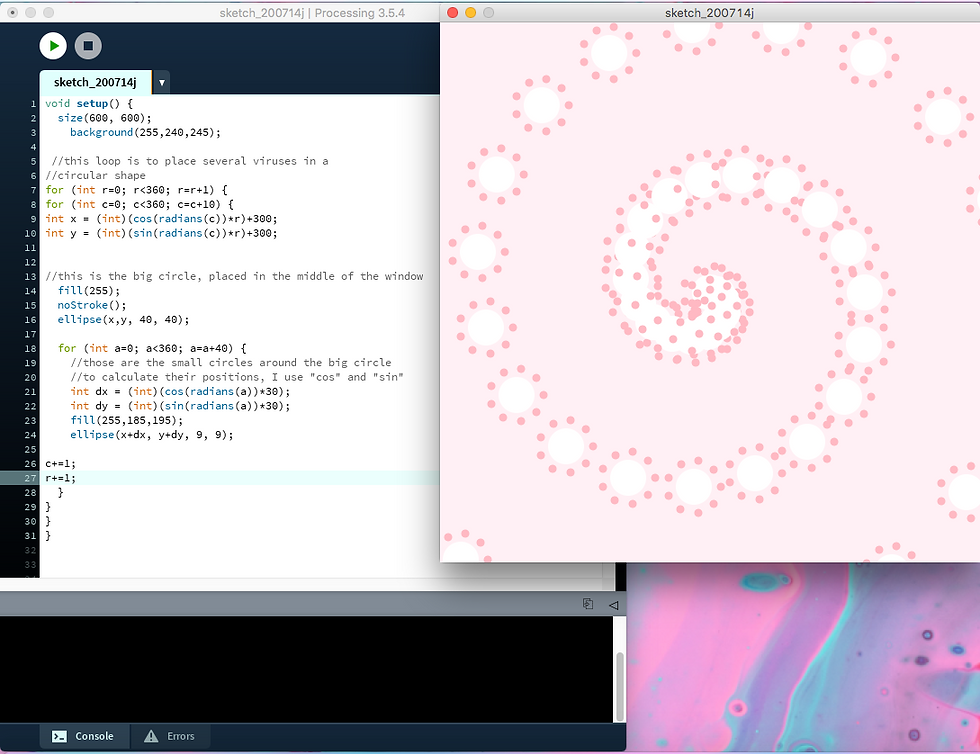
This is the code:
void setup() {
size(600, 600);
background(255,240,245);
//this loop is to place several viruses in a
//circular shape
for (int r=0; r<360; r=r+1)This increases the distance between groups of circle {
for (int c=0; c<360; c=c+10)This makes the spiral look, if we increase the value to for example c=c+50 it looks like the last excercise {
int x = (int)(cos(radians(c))*r)+300;
int y = (int)(sin(radians(c))*r)+300;
//this is the big circle, placed in the middle of the window
fill(255);
noStroke();
ellipse(x,y, 40, 40);
for (int a=0; a<360; a=a+40) {
//those are the small circles around the big circle
//to calculate their positions, I use "cos" and "sin"
int dx = (int)(cos(radians(a))*30);
int dy = (int)(sin(radians(a))*30);
fill(255,185,195);
ellipse(x+dx, y+dy, 9, 9);
c+=1;
r+=1;
These are very imporant values, since they determine the distance between the groups of circle, in a radius way
}
}
}
}
Comments